How To Fix Styled Components Page Flicker in Next.js 12+
July 2, 2022
1 min
If you're seeing flickering styles and layout shifts while using Styled Components with Next.js 12+, there is a quick and simple solution to fixing this. Most of the articles you'll read are fixes for Next.js version 11 and below, so try this out if none of the other solutions are working for you.
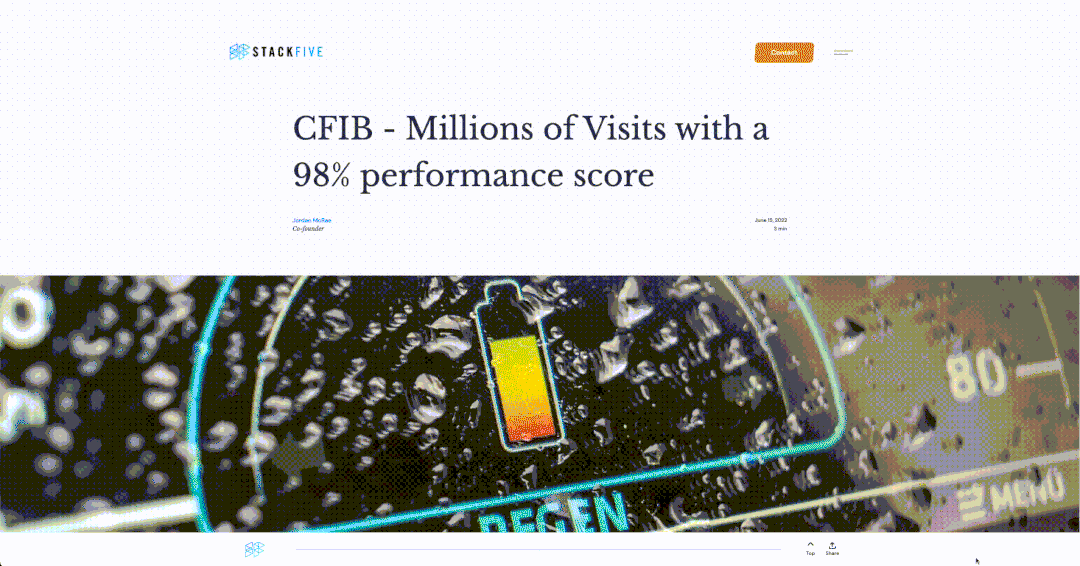
An example of some annoying page flickering when the page loads
The Code
1. In your next.config.js file, add this:
1const nextConfig = {
2 reactStrictMode: true,
3 compiler: {
4 styledComponents: true,
5 }
6};
7
8module.exports = nextConfig;
9
2. If you don't already have one, create a _document.js
file in your pages
folder with this:
1import Document from 'next/document'
2import { ServerStyleSheet } from 'styled-components'
3
4export default class MyDocument extends Document {
5 static async getInitialProps(ctx) {
6 const sheet = new ServerStyleSheet()
7 const originalRenderPage = ctx.renderPage
8
9 try {
10 ctx.renderPage = () =>
11 originalRenderPage({
12 enhanceApp: (App) => (props) =>
13 sheet.collectStyles(<App {...props} />),
14 })
15
16 const initialProps = await Document.getInitialProps(ctx)
17 return {
18 ...initialProps,
19 styles: [initialProps.styles, sheet.getStyleElement()],
20 }
21 } finally {
22 sheet.seal()
23 }
24 }
25}
3. Because of the compiler changes in Next.js 12+, you no longer need to use the Babel configuration for Styled Components. Make sure you don’t have anything related to Styled Components in your .babelrc file (if you have one).
And that’s all there is to it! You should no longer have page flicker in your development and production environments.
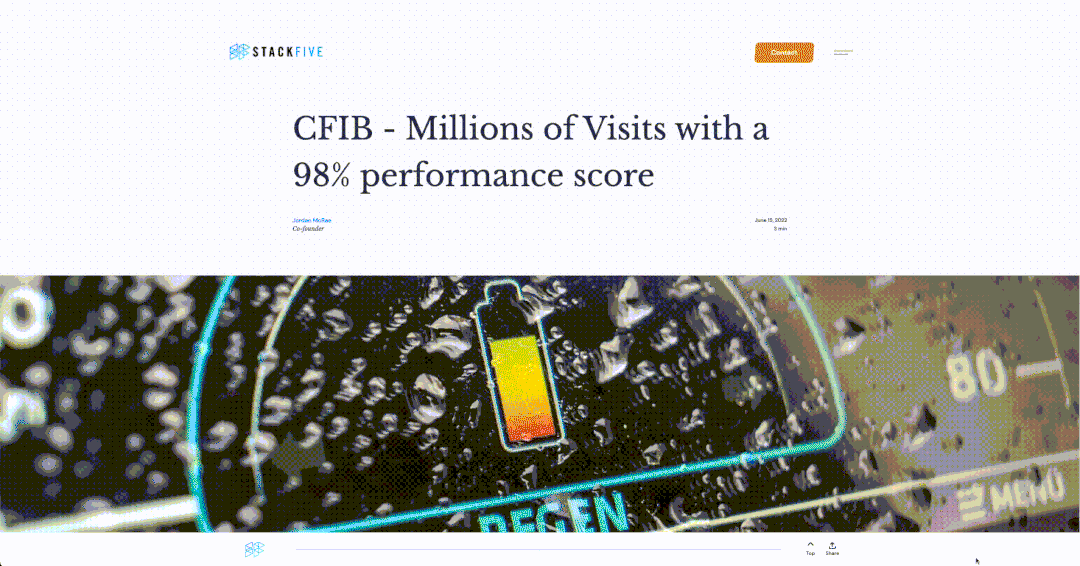
Before
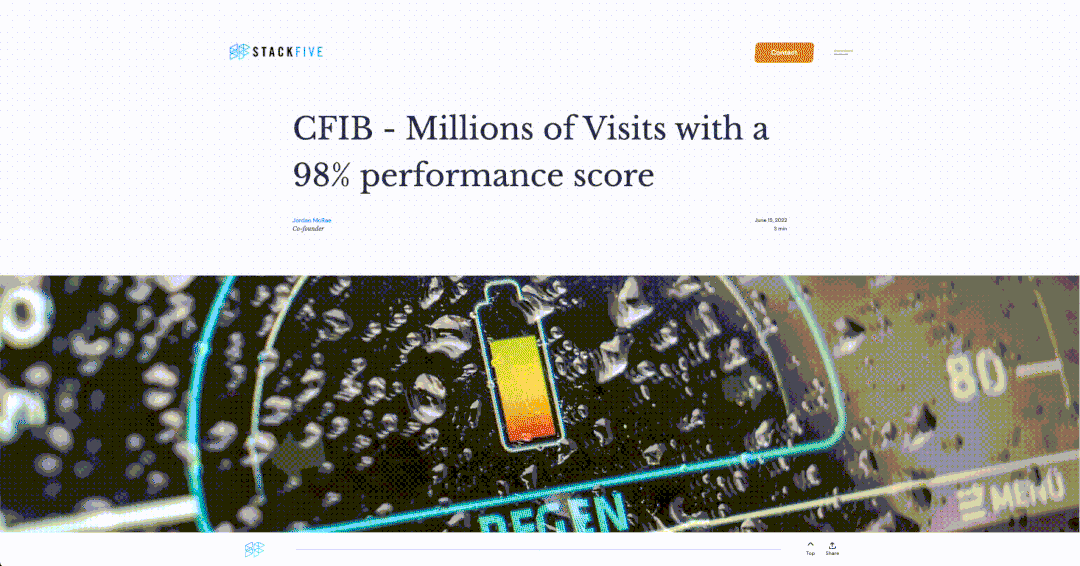
After
We're Here to Help
Do you need some assistance with your Next.js application, or have a digital product you're interested in building with Next.js? Please reach out to our team, and we'd love to chat. We use Next.js for many of our custom production web applications because Next.js is such a powerful and scalable tool.