Seeding Relational Data with Prisma
August 8, 2022
2 min
Prisma is a super simple ORM for your SQL databases. Let's run through how we seed relational data with it. You can also check out the sample app on Github.
Our Schema
Let's assume your Prisma.schema file looks like this:
1datasource db {
2 provider = "postgresql"
3 url = env("DATABASE_URL")
4}
5
6generator client {
7 provider = "prisma-client-js"
8}
9
10model User {
11 id String @id @default(uuid())
12 createdAt DateTime @default(now())
13 updatedAt DateTime @updatedAt
14 posts Post[]
15}
16
17model Post {
18 id String @id @default(uuid())
19 createdAt DateTime @default(now())
20 updatedAt DateTime @updatedAt
21 text String
22 user User @relation(fields: [userId], references: [id])
23 userId String
24}
Here we have a User model that can have many Posts, and a Post belongs to a User.
Seeding Our Dev Database
Assuming you've got Prisma set up already (if you don't, check out the docs), let's create some dummy data for our development environment.
- Inside of your project's prisma folder, create a new folder called seed. This is where we will set up our seeding program, as well as our dummy data.
- Inside of your seed folder, create a new file called index.js, and a new folder called data.
- Inside of your data folder, create two files: users.js and posts.js.
Your folder structure should look something like this:
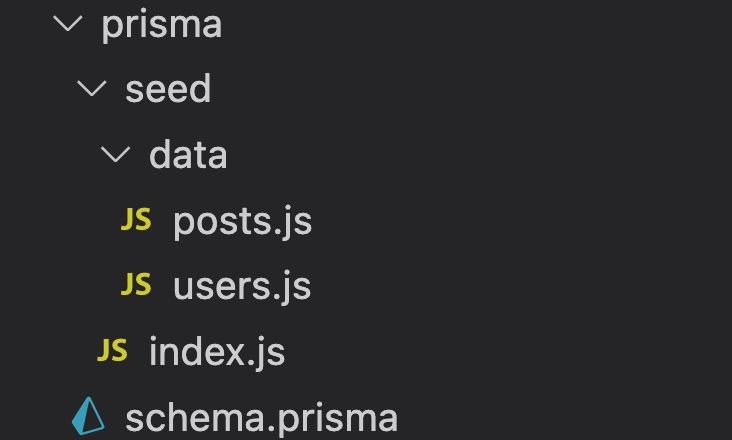
What your folder structure should look like
Inside of your users.js file, let's create two dummy users that match our schema:
1module.exports = [
2 // User 1
3 {
4 id: 'f1bdf45e-1b1c-11ec-9621-0242ac130002',
5 createdAt: new Date(
6 'Tue Sep 21 2021 16:16:50 GMT-0400 (Eastern Daylight Time)'
7 ),
8 updatedAt: new Date(
9 'Tue Sep 21 2021 16:16:50 GMT-0400 (Eastern Daylight Time)'
10 ),
11 },
12
13 // User 2
14 {
15 id: '9371f314-1c93-11ec-9621-0242ac130002',
16 createdAt: new Date(
17 'Tue Sep 21 2021 16:16:50 GMT-0400 (Eastern Daylight Time)'
18 ),
19 updatedAt: new Date(
20 'Tue Sep 21 2021 16:16:50 GMT-0400 (Eastern Daylight Time)'
21 ),
22 }
23];
Inside of your posts.js file, let's create a post for each user.
Note: in the userId field for each post, you'll need to use a user's id from the users.js file we created in order to link up the relationship:
1module.exports = [
2 // User 1's Post
3 {
4 id: '8e3399e6-1d94-11ec-9621-0242ac130002',
5 createdAt: new Date(
6 'Tue Sep 21 2021 16:16:50 GMT-0400 (Eastern Daylight Time)'
7 ),
8 updatedAt: new Date(
9 'Tue Sep 21 2021 16:16:50 GMT-0400 (Eastern Daylight Time)'
10 ),
11 text: 'Lorem ipsum dolor sit amet, consectetur adipiscing elit. Praesent sed.',
12 userId: 'f1bdf45e-1b1c-11ec-9621-0242ac130002',
13 },
14
15 // User 2's Post
16 {
17 id: '9197dea8-1d94-11ec-9621-0242ac130002',
18 createdAt: new Date(
19 'Tue Sep 21 2021 16:16:50 GMT-0400 (Eastern Daylight Time)'
20 ),
21 updatedAt: new Date(
22 'Tue Sep 21 2021 16:16:50 GMT-0400 (Eastern Daylight Time)'
23 ),
24 text: 'Lorem ipsum dolor sit amet, consectetur adipiscing elit. Praesent sed.',
25 userId: '9371f314-1c93-11ec-9621-0242ac130002',
26 }
27];
Inside of the prisma/seed/index.js file is where the magic happens. This is where we'll write our code to tell Prisma what to do with the dummy data we just wrote. Inside of your index.js file, let's use the following code:
1const { PrismaClient } = require('@prisma/client');
2
3const prisma = new PrismaClient();
4
5const Users = require('./data/users');
6const Posts = require('./data/Posts');
7
8async function runSeeders() {
9 // Users
10 await Promise.all(
11 Users.map(async (user) =>
12 prisma.user.upsert({
13 where : { id: user.id },
14 update: {},
15 create: user,
16 })
17 )
18 );
19
20 // Posts
21 await Promise.all(
22 Posts.map(async (post) =>
23 prisma.post.upsert({
24 where: { id: post.id },
25 update: {},
26 create: post,
27 })
28 )
29 );
30}
31
32runSeeders()
33 .catch((e) => {
34 console.error(`There was an error while seeding: ${e}`);
35 process.exit(1);
36 })
37 .finally(async () => {
38 console.log('Successfully seeded database. Closing connection.');
39 await prisma.$disconnect();
40 });
And the last step is to run our seed utility! Inside of your package.json file, create a new "script" entry:
1{
2 "scripts": {
3 "seed": "prisma db push && node prisma/seed/index.js"
4 }
5}
What this command is doing is telling Prisma to sync up your database with the models you defined in your schema, and then running our seed utility.
You can now run this with either yarn seed
or npm run seed
!
Once it completes, you can check out your data using the command prisma studio
.
And voila! You've successfully seeded some relational data. If you'd like to try it yourself, you can check out the sample app on Github.